Claims-Based Authorization
It is generally agreed by many
that roles are sufficient for simple cases where the services do not
need to provide fine-grained access control over resource and
operations. Claims-Based Authorization [MPP] goes a step beyond the use
of roles to govern resource access. Many times roles do not provide
enough precision to enable the control that you might need for a given
service. Claims, however, are able to represent many characteristics
needed for different security scenarios, including identity, roles,
rights, and other attributes of the consumer that might be of interest
to the service.
A
claim can be literally anything that describes aspects of a subject, be
it an actual person or an abstract resource. A claim is endorsed by an
authority; which means one observer can decide if the fact the claim
represents should be considered true according to the authority’s
trustworthiness.
For example, the passports
we use as a form of identification when traveling outside of our
resident countries can be considered as a form of claims-based identity.
The passport document represents a token (typically issued and signed
by authoritative government agencies), which contains information (or
specifically, claims) such as ID number, name, birth date, and other
aspects that are associated with an individual.
The passport allows us to enter
other countries (security domains) because of an existing trust
relationship. The process of supplying credentials to prove or verify
our identity only needs to be done once with the passport-issuing agency
(as with a Security Token Service). We no longer need to
re-authenticate again once we have a valid passport; we just need to
present it as a form of trusted token.
|
A claim represents a right with
respect to a particular value. The right can be considered an action,
such as read and write, or it can posses a value that can be viewed as
some system resource, such as a database, a file, or an operation.
Claims are governed by claim type, which is a value representing a
category of claims. For example, a service consumer could present a
claim of type file with a read right over a value of “resume.doc.” This
translates to a read permission to the file “resume.doc.”
Claims are generic enough to
enable rights to be exercised over many different types of objects. For
example, an internal consumer of a service might possess a Windows
credential as proof of identity, whereas an external consumer accessing
the same service may not have a Windows credential that can be validated
by the service. The service may therefore require X.509 certificates
for these consumers. In either case, the consumers can assert their
identities using claims.
WCF generates claims from security tokens. Security tokens are
abstractions of credentials validated by the security policy of the
service that populates the security headers of an incoming message. Once
the security token is validated,
the claims are extracted and placed in the security context of the
current operation. The resultant set of claims is known as a claim set. A claim set is a collection of claims provided by a common issuer and exists for each set of credentials being authenticated.
The authorization manager
allows claim sets to be added to the security context of a request.
Claim sets can be created from the security token passed to the service
in the message. The goal of the claims created in this manner is to
provide a unified mechanism for evaluating the information found in many
disparate types of security tokens. The framework extracts the evidence
found in these security tokens and represents it as a claim set.
Claim sets can also be added
to context administratively. This feature allows additional claims to be
added to the security context allowing the local security policy to be
represented in the claim set.
The service authorization
manager makes the final decision to grant or deny the calling consumer
program access to the service’s resources. The role of the service
authorization manager is to determine the access requirements of the
resource in question and inspect the evidence presented by the consumer
to determine whether it meets those requirements. The decision is made
to grant or deny access based on this evaluation.
Claims Processing in WCF
Claims are a first class citizen of WCF and are represented by the Claim
type. A claim represents an assertion by a consumer that it has a right
to the requested resource. That resource might be possession of an
identity or permission, such as read access to a database. Claims can be
constructed at runtime by creating an instance of the Claim class and setting its Type, Resource and Right properties, as follows:
Example 7.
Claim emailClaim = new Claim (ClaimTypes.Email, "[email protected]", Rights.PossessProperty);
|
As mentioned previously,
a claim set represents a collection of claims issued by the same
source. A claim set extracted from a CardSpace token would be placed in
the security context with the CardSpace provider identified as the
issuer. The Issuer member of ClaimSet is another ClaimSet that represents the issuer of the consumer program’s claims.
ClaimSet is an abstract class, meaning you cannot explicitly create an instance of ClaimSet. Instead, you must use the DefaultClaimSet type or create a new type derived from ClaimSet and create an instance of that instead. It is important to note that once the claim set is created, it cannot be changed.
The WCF service model
processes the security tokens provided in the message and creates claim
sets based on the type of token being processed. The generated claims
are placed in the security context and become accessible at runtime
through the AuthorizationContext.
AuthorizationContext contains a quantity of ClaimSet equal to the number of security tokens in the request and the number of authorization policies associated with the service.
Accessing claims from the authorization context is quite straightforward as shown here:
Example 8.
AuthorizationContext context = ServiceSecurityContext.Current.AuthorizationContext; foreach(ClaimSet claims in context.ClaimSets) { WindowsClaimSet windowsClaims; if(claims.GetType() == typeof(WindowsClaimSet)) { windowsClaims = (WindowsClaimSet)claims; if(windowsClaims.ContainsClaim(new Claim (ClaimTypes.Email, "[email protected]", Rights.PossessProperty)) == false) throw new Exception("It's not who we want it to be!"); } }
|
Implementing Claims-Based Authorization
The value of a claims-based
model is its ability to represent consumer assertions in a consistent
manner, regardless of the source of the assertion. Most services need to
support consumers regardless of domain or platform affiliation.
Authorizing resource access can prove exceptionally difficult without a
means to normalize consumer credentials and security demands. Rather
than restrict the service to a limited use model (such as roles), we can
design a claims-based system that enables us to build a flexible
authorization mechanism that is not bound to a specific credential type
or collection of roles.
In
this way the service can meet the authorization requirements of the
consumer designers without affecting business requirements tied to
consumer identities (Figure 3).
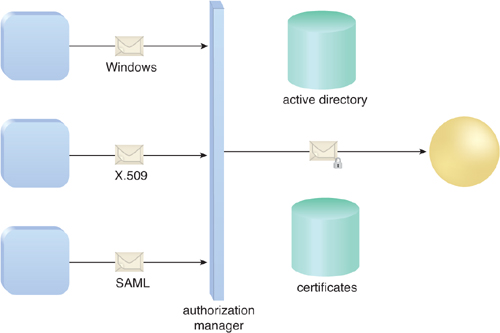
Access Control in Windows Azure
With Windows Azure
services, authorization implementations and integrations can be
externalized from the service logic and managed as a set of declarative
rules through the use of Access Control. Windows Azure Access Control
supports claims-based authorizations in federated identity scenarios,
enabling single sign-on across separate security domains.
For example, organizations that leverage Active Directory Federation Services (explained shortly in the Windows Identity Foundation (WIF)
section) can enable users/consumers to authenticate and sign on to
external cloud-based services using the organization’s identities.
Access Control features include:
claims transformation engine using declarative rules and policies
security token service (STS)
support for multiple credentials, including Windows Live IDs, SAML tokens, and X.509 certificates
setup Issuer trust with a simple Web interface or programmatically through APIs
support for Active Directory and other identity infrastructures
Designing Custom Claims
As
is the case with any architectural approach to security, the first step
is to determine which resources need to be protected and the
requirements necessary to access those resources. The result of this
activity becomes the basis of custom claims designed for the service.
Often times, a
resource-centric security model is the most straightforward approach to
take. The goal of service security is to protect the resources under the
care of the service implementation. Resources can be data (such as a
business entity) or a physical resource (such as a report). Consumer
rights can be established by defining a specific set of actions that can
be performed on those resources.