The Libraries folder provided with Windows
7 holds user-defined collections of resources. Placing projects, files,
pictures, sounds, and other items in this folder means that the user
can find them with ease, organize them, search within them, and
generally access the things needed to conduct business, without having
to consider where the resource is actually located. The following
sections describe how to interact with the user-defined collections.
1. Configuring the User-Defined Collection Example
This example begins with a Windows Forms application. You'll need to add a List button (btnList) to obtain a list of the known folders and their content, an Add button (btnAdd) to add a new item to the collection, and a list box (lstItems) to list the contents of the user-defined collection. In addition, you'll need to add a reference to Microsoft.WindowsAPICodePack.Shell.DLL and provide the following using statement:
using Microsoft.WindowsAPICodePack.Shell;
Because this example also needs a special folder to
add to the library you're creating, you need to create it. For the
purpose of this example, use Windows Explorer to create a C:\Example folder. In the C:\Example folder, create a text file named Temp.TXT. You don't need to worry about any content; you won't be using it in this case.
2. Listing Libraries
The default Libraries folder contains four items:
Documents, Music, Pictures, and Videos. However, it can contain any
number of items, depending on what the user chooses to add to it. A
user adds an item to the Libraries folder by right-clicking any open
area and choosing New
Library from the Context menu. When the user opens the library, it asks
the user to start adding folders to it. Unlike general purpose folders,
a user can also optimize a library to hold specific types of
information such as music or pictures. In short, a library is a special
kind of folder.
The User-Defined Collection example performs two
tasks. First, it lists the content of the Libraries folder. Second, it
adds a new library to the folder. These two tasks represent the kinds
of things you'll normally do programmatically. An application can add
special entries to the Libraries folder to do things like make project
handling automatic or help the user automate the optimizing of library
content. Listing 1 shows the code needed to perform the first task, listing the library content.
Example 1. Listing the user-defined collections
private void btnList_Click(object sender, EventArgs e) { // Clear the old entries. lstItems.Items.Clear();
// Obtain access to the Libraries folder.
IKnownFolder Libraries = KnownFolders.UsersLibraries;
// Output the individual library entries. foreach (ShellLibrary Library in Libraries) { // Display information about each library. lstItems.Items.Add("Name: " + Library.Name); lstItems.Items.Add("Default Save Folder: " + Library.DefaultSaveFolder); lstItems.Items.Add("Is File System Object? " + Library.IsFileSystemObject); lstItems.Items.Add("Is Link? " + Library.IsLink); lstItems.Items.Add("Is Pinned to Navigation Pane? " + Library.IsPinnedToNavigationPane); lstItems.Items.Add("Is Read-Only? " + Library.IsReadOnly); lstItems.Items.Add("Library Type: " + Library.LibraryType); lstItems.Items.Add("Library Type ID: " + Library.LibraryTypeId);
// Display a list of folders in the library. lstItems.Items.Add("Folder List:"); foreach (ShellFileSystemFolder Folder in Library) lstItems.Items.Add("\t" + Folder.Name);
// Add a blank line between entries. lstItems.Items.Add(""); } }
|
Each of the types handled by KnownFolders has different capabilities and properties — the ShellLibrary type is no different. Every entry in KnownFolders.UsersLibraries is of the ShellLibrary type, so you can use that type directly when enumerating the user-defined collections.
The ShellLibrary type does contain familiar properties such as Name and IsFileSystemObject. However, notice some of the differences in this case, such as IsPinnedToNavigationPane and LibraryType. These properties make sense only when working with a library entry. Especially important is the LibraryType because it tells you what kind of data the user expects to see in that library.
A library will also contain some type of content or it isn't useful. The top-level content is always a list of ShellFileSystemFolder items (folders). A user always adds an entire folder, not individual files, to a library. Each of the ShellFileSystemFolder entries can contain a mix of folders and files. Figure 1 shows typical output from this example.
3. Adding Libraries
At some point you'll probably want to create some
type of custom library for the user, especially if your application
supports projects. The application can create a project library that
gathers all the resources for the project into one place. The user need
not know where any of these resources are stored (and probably doesn't
care to know either). Adding a library is straightforward, as shown in Listing 1.
Example 1. Adding a new library
private void btnAdd_Click(object sender, EventArgs e) { // Create a new library. ShellLibrary NewLibrary = new ShellLibrary("A New Library", true);
// Add a folder to the new library. NewLibrary.Add(@"C:\Example");
// Define the library type. NewLibrary.LibraryType = LibraryFolderType.Documents;
// Close the library when finished. NewLibrary.Close();
// Tell the user the library is added. MessageBox.Show("Library Successfully Added!"); }
|
There are a number of ways to create a new library, but the most common method is to define a new ShellLibrary object, NewLibrary, and specify a name for it using the first ShellLibrary() constructor argument. The second ShellLibrary()
constructor argument specifies whether the application can overwrite an
existing library of the same name. Because you're apt to run this
example multiple times and the library doesn't contain any real data,
it's just fine to overwrite it. In a real-world application, you'll
probably set this second argument to false to avoid overwriting valuable information.
A library isn't much good without content. The example adds the folder you created earlier to NewLibrary using the Add() method. You can add as many folders as needed using this approach. The Add()
method provides several overrides so you can add folders of various
types, but remember that you can add only folders to a library, not
files.
It's important to optimize a library whenever possible. The most basic optimization is to set the LibraryType property using a LibraryFolderType enumeration member. The example optimizes the library we've created to hold documents.
Always close a library before you exit the method
that creates it, even if you have to reopen it later. Otherwise, it's
possible you could lose some of the library configuration information.
Once the library is added, you can see it in the Libraries folder, as
shown in Figure 2.
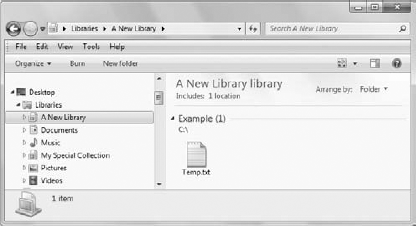