The Jupiter Motor's ERP system database and the Dealer Orders database
Here is a visual representation of our databases. The following is the visual look at the portal database:
The following is the visual look of the Jupiter ERP database:
The portal database design is
very simple, consisting of only five tables. The Jupiter ERP database
has only three tables, and will be a local database (this will not live
in SQL Azure; it is a representation of an on-site ERP system). Now that
we know what the designs of the databases are, let's get started with
the database creation of the portal database in SQL Azure.
SQL Azure portal
When we sign up for the SQL Azure service at http://windows.azure.com,
we are given a URL to the Azure portal. To access our SQL Azure area of
the portal, click on the link in the menu bar to the left for SQL
Azure, as seen here:
There is some basic information such as the Server Name (the URL is for connections from external sources, such as SSMS), the Administrator Username, and our selected Server Location,
which we will use throughout our travels in SQL Azure. Also, in the box
at the bottom we can see a visual list of our databases (including the
default master database that was already created for us). We are also
able to change firewall settings to let specific IP blocks into our SQL
Azure service. We can also create a new database here, view connection
strings to the selected database, test connections to the database, or
drop a database.
To use SQL Azure
from an outside connection via a tool such as SSMS, we must whitelist
the IP block we are connecting from. To do this, select the Firewall Settings tab and click the Add Rule
button. A popup opens that allows us to enter IP range. To make things a
little easier, our current IP address is displayed. When finished,
click the Submit button; it may take up to five minutes for the changes to propagate.
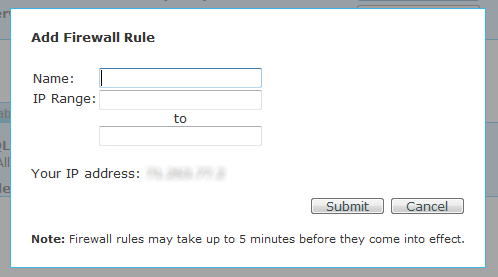
Creating our database
Now that we've done everything
we need to do to hit our SQL Azure database from an outside connection,
let's set up the database by using the Server URL in SSMS and our
Administrator Username/Password to connect from there. Once connected,
the following scripts will create our application databases, add logins
named JupiterMotors for the JupiterERP database and Portal for the Portal
database, and all the
tables/keys in the diagram at the beginning of the article . Please note
that the CREATE DATABASE and CREATE LOGIN commands must be executed separately in the master database, and the CREATE USER
command is to be executed in the Jupiter ERP database. Also, we must
log into the each database through SSMS to run the script for creating
the tables and keys.
We will first start with creating the portal database, login, and user:
/* Execute this line in the master database */
CREATE DATABASE [Portal]
/* Execute this line in the master database */
CREATE LOGIN Portal WITH Password='P@ssword'
/* Execute this line in the Portal database */
CREATE USER Portal FROM LOGIN Portal
Next, we're going to create the tables. This code must be executed after logging directly into the portal database using SSMS:
/*********************************************************************************
* This section below will create our tables for the Portal database
* -Customers table
* This table will hold all of our Customer address information
* -OrderStauuses table
* This table will hold all of our Order Statuses for our orders
* -OrderHeaders table
* This table will hold our Order Header information
* -OrderDetails table
* This table will hold our Order Detail information
* -OrderPictures table
* This table will hold the information of the pictures placed into
* Blob Storage for the Jupiter Motors portal
*********************************************************************************/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
SET ANSI_PADDING ON
databasecreatingGO
CREATE TABLE [dbo].[Customers](
[CustomerID] [int] IDENTITY(1,1) NOT NULL,
[CustomerName] [varchar](50) NOT NULL,
[CustomerAddress1] [varchar](50) NOT NULL,
[CustomerAddress2] [varchar](50) NULL,
[City] [varchar](50) NOT NULL,
[StateProv] [varchar](50) NOT NULL,
[PostalCode] [varchar](50) NOT NULL,
CONSTRAINT [PK_Customers] PRIMARY KEY CLUSTERED
(
[CustomerID] ASC
))
GO
CREATE TABLE [dbo].[OrderStatuses](
[OrderStatusId] [int] IDENTITY(1,1) NOT NULL,
[Description] [varchar](50) NOT NULL,
CONSTRAINT [PK_OrderStatuses] PRIMARY KEY CLUSTERED
(
[OrderStatusId] ASC
))
GO
CREATE TABLE [dbo].[OrderHeaders](
[OrderHeaderID] [int] IDENTITY(1,1) NOT NULL,
[OrderDate] [smalldatetime] NOT NULL,
[RequestedDate] [smalldatetime] NOT NULL,
[CustomerPO] [varchar](50) NOT NULL,
[CustomerID] [int] NOT NULL,
[OrderStatusID] [int] NOT NULL,
CONSTRAINT [PK_OrderHeader] PRIMARY KEY CLUSTERED
(
[OrderHeaderID] ASC
))
GO
CREATE TABLE [dbo].[OrderPictures](
[OrderPictureID] [int] IDENTITY(1,1) NOT NULL,
[OrderHeaderID] [int] NOT NULL,
[PictureFile] [varchar](100) NOT NULL,
[PictureDate] [smalldatetime] NOT NULL,
CONSTRAINT [PK_OrderPictures] PRIMARY KEY CLUSTERED
(
[OrderPictureID] ASC
))
GO
databasecreatingCREATE TABLE [dbo].[OrderDetails](
[OrderDetailID] [int] IDENTITY(1,1) NOT NULL,
[OrderHeaderID] [int] NOT NULL,
[ItemNumber] [int] NOT NULL,
[ItemDescription] [nchar](10) NULL,
[QuantityOrdered] [int] NOT NULL,
CONSTRAINT [PK_OrderDetails] PRIMARY KEY CLUSTERED
(
[OrderDetailID] ASC
))
GO
After the tables have been created, let's now create the database keys:
/*********************************************************************************
* This section below will create our foreign keys for the Portal database
* -FK_OrderDetails_OrderHeaders
* Links OrderDetails to OrderHeaders using OrderHeaderID Primary Key
* -FK_OrderHeaders_Customers
* Links OrderHeaders to Customers using CustomerID Primary Key
* -FK_OrderPictures_OrderHeaders
* Links OrderPictures to OrderHeaders using OrderHeaderID Primary Key
* -FK_OrderHeaders_OrderStatuses
* Links OrderHeaders to OrderStatuses using OrderStatusID Primary Key
*********************************************************************************/
ALTER TABLE [dbo].[OrderDetails] WITH CHECK ADD CONSTRAINT [FK_OrderDetails_OrderHeaders] FOREIGN KEY([OrderHeaderID])
REFERENCES [dbo].[OrderHeaders] ([OrderHeaderID])
GO
ALTER TABLE [dbo].[OrderDetails] CHECK CONSTRAINT [FK_OrderDetails_OrderHeaders]
GO
ALTER TABLE [dbo].[OrderPictures] WITH CHECK ADD CONSTRAINT [FK_OrderPictures_OrderHeaders] FOREIGN KEY([OrderHeaderID])
REFERENCES [dbo].[OrderHeaders] ([OrderHeaderID])
GO
ALTER TABLE [dbo].[OrderPictures] CHECK CONSTRAINT [FK_OrderPictures_OrderHeaders]
GO
ALTER TABLE [dbo].[OrderHeaders] WITH CHECK ADD CONSTRAINT [FK_OrderHeaders_Customers] FOREIGN KEY([CustomerID])
REFERENCES [dbo].[Customers] ([CustomerID])
GO
ALTER TABLE [dbo].[OrderHeaders] CHECK CONSTRAINT [FK_OrderHeaders_Customers]
GO
ALTER TABLE [dbo].[OrderHeaders] WITH CHECK ADD CONSTRAINT [FK_OrderHeaders_OrderStatuses] FOREIGN KEY([OrderStatusID])
REFERENCES [dbo].[OrderStatuses] ([OrderStatusId])
GO
ALTER TABLE [dbo].[OrderHeaders] CHECK CONSTRAINT [FK_OrderHeaders_OrderStatuses]
GO
Finally, for our Portal database, we must create the stored procedures to insert customers, order headers, and order detail:
/*********************************************************************************
* This section below will create our stored procedures in the
* Portal database. There is one stored procedure for creating
* the new customer placing the order, one to insert the Order Header, and one
* that will insert each line item on the order into the Order Details table.
* Orders will be inserted with a OrderStatusID = 1 (Unapproved) in the
* OrderHeaders table.
*********************************************************************************/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE PROCEDURE [dbo].[NewCustomer]
@Name varchar(50),
@Address1 varchar(50),
@Address2 varchar(50),
@City varchar(50),
@StateProv varchar(50),
@PostalCode varchar(50)
AS
BEGIN
SET NOCOUNT ON;
INSERT INTO Customers(CustomerName, CustomerAddress1, CustomerAddress2, City, StateProv, PostalCode)
VALUES(@Name, @Address1, @Address2, @City, @StateProv, @PostalCode)
SELECT Scope_Identity()
END
databasecreatingGO
CREATE PROCEDURE [dbo].[NewOrderHeader]
@OrderDate datetime,
@RequestedDate datetime,
@CustomerPO varchar(50),
@CustomerID int
AS
BEGIN
SET NOCOUNT ON;
INSERT INTO OrderHeaders(OrderDate, RequestedDate, CustomerPO, CustomerID, OrderStatusID)
VALUES (@OrderDate, @RequestedDate, @CustomerPO, @CustomerID, 1)
SELECT SCOPE_IDENTITY()
END
GO
CREATE PROCEDURE [dbo].[NewOrderDetail]
@OrderHeaderID int,
@ItemNumber varchar(50),
@ItemDescription varchar(50),
@QuantityOrdered int
AS
BEGIN
SET NOCOUNT ON;
INSERT INTO OrderDetails(OrderHeaderID, ItemNumber, ItemDescription, QuantityOrdered)
VALUES(@OrderHeaderID, @ItemNumber, @ItemDescription, @QuantityOrdered)
END
GO
Our portal maintains the order status in the OrderHeaders table. Let's insert the statuses in the OrderStatuses table:
/**************************************************************
databasecreating* These statements will insert the Order Statuses
**************************************************************/
INSERT INTO dbo.OrderStatuses(Description)
VALUES ('Unapproved')
INSERT INTO dbo.OrderStatuses(Description)
VALUES ('Approved')
INSERT INTO dbo.OrderStatuses(Description)
VALUES ('Scheduled For Production')
INSERT INTO dbo.OrderStatuses(Description)
VALUES ('In Production')
INSERT INTO dbo.OrderStatuses(Description)
VALUES ('In Transit for Delivery')
INSERT INTO dbo.OrderStatuses(Description)
VALUES ('Accepted')
INSERT INTO dbo.OrderStatuses(Description)
VALUES ('Complete')
That takes care of the Portal database. We will now create the JupiterERP
database. This creation of database, logins, users, tables, keys, and
stored procedures use the same steps as creating the Portal database
above, only the database structure is much simpler and this database
will reside on our physical machine. Remember that this database is
stored on a local instance of SQL Server, so we have our full set of
features with this database (though the scripts were written with the
simplest features for ease):
/* Execute this line in the master database */
CREATE DATABASE [JupiterERP]
/* Execute this line in the master database */
CREATE LOGIN JupiterMotors WITH Password='P@ssword'
/* Execute this line in the JupiterERP database */
CREATE USER JupiterMotors FROM LOGIN JupiterMotors
The creation script for the tables and keys follows (remember to connect directly to the local instance of our JupiterERP database to run this script and the stored procedures script):
/*********************************************************************************
databasecreating* This section below will create our tables for the JupiterERP database
* -Customers table
* This table will hold all of our Customer address information
* -OrderHeaders table
* This table will hold our Order Header information
* -OrderDetails table
* This table will hold our Order Detail information
*********************************************************************************/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
SET ANSI_PADDING ON
GO
CREATE TABLE [dbo].[Customers](
[CustomerID] [int] IDENTITY(1,1) NOT NULL,
[CustomerName] [varchar](50) NOT NULL,
[CustomerAddress1] [varchar](50) NOT NULL,
[CustomerAddress2] [varchar](50) NULL,
[City] [varchar](50) NOT NULL,
[StateProv] [varchar](50) NOT NULL,
[PostalCode] [varchar](50) NOT NULL,
CONSTRAINT [PK_Customers] PRIMARY KEY CLUSTERED
(
[CustomerID] ASC
))
CREATE TABLE [dbo].[OrderHeaders](
[OrderHeaderID] [int] IDENTITY(1,1) NOT NULL,
[OrderDate] [smalldatetime] NOT NULL,
[RequestedDate] [smalldatetime] NOT NULL,
[CustomerPO] [varchar](50) NOT NULL,
[CustomerID] [int] NOT NULL,
CONSTRAINT [PK_OrderHeader] PRIMARY KEY CLUSTERED
(
[OrderHeaderID] ASC
))
CREATE TABLE [dbo].[OrderDetails](
[OrderDetailID] [int] IDENTITY(1,1) NOT NULL,
[OrderHeaderID] [int] NOT NULL,
[ItemNumber] [int] NOT NULL,
[ItemDescription] [nchar](10) NULL,
[QuantityOrdered] [int] NOT NULL,
CONSTRAINT [PK_OrderDetails] PRIMARY KEY CLUSTERED
(
[OrderDetailID] ASC
))
GO
databasecreating/*********************************************************************************
* This section below will create our foreign keys for the JupiterERP database
* -FK_OrderDetails_OrderHeaders
* Links OrderDetails to OrderHeaders using OrderHeaderID Primary Key
* -FK_OrderHeaders_Customers
* Links OrderHeaders to Customers using CustomerID Primary Key
*********************************************************************************/
ALTER TABLE [dbo].[OrderDetails] WITH CHECK ADD CONSTRAINT [FK_OrderDetails_OrderHeaders] FOREIGN KEY([OrderHeaderID])
REFERENCES [dbo].[OrderHeaders] ([OrderHeaderID])
GO
ALTER TABLE [dbo].[OrderDetails] CHECK CONSTRAINT [FK_OrderDetails_OrderHeaders]
GO
ALTER TABLE [dbo].[OrderHeaders] WITH CHECK ADD CONSTRAINT [FK_OrderHeaders_Customers] FOREIGN KEY([CustomerID])
REFERENCES [dbo].[Customers] ([CustomerID])
GO
ALTER TABLE [dbo].[OrderHeaders] CHECK CONSTRAINT [FK_OrderHeaders_Customers]
GO
The last thing to do is to create the stored procedures to add a customer, an order header, and the order detail:
/*********************************************************************************
databasecreating* This section below will create our stored procedures in the
* JupiterERP database. There is one stored procedure for creating
* the new customer placing the order, one to insert the Order Header, and one
* that will insert each line item on the order into the Order Details table.
*********************************************************************************/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE PROCEDURE [dbo].[NewCustomer]
@Name varchar(50),
@Address1 varchar(50),
@Address2 varchar(50),
@City varchar(50),
@StateProv varchar(50),
@PostalCode varchar(50)
AS
BEGIN
SET NOCOUNT ON;
INSERT INTO Customers(CustomerName, CustomerAddress1, CustomerAddress2, City, StateProv, PostalCode)
VALUES(@Name, @Address1, @Address2, @City, @StateProv, @PostalCode)
SELECT SCOPE_IDENTITY()
END
GO
CREATE PROCEDURE [dbo].[NewOrderHeader]
@OrderDate datetime,
@RequestedDate datetime,
@CustomerPO varchar(50),
@CustomerID int
AS
BEGIN
SET NOCOUNT ON;
INSERT INTO OrderHeaders(OrderDate, RequestedDate, CustomerPO, CustomerID)
VALUES (@OrderDate, @RequestedDate, @CustomerPO, @CustomerID)
SELECT SCOPE_IDENTITY()
END
GO
CREATE PROCEDURE [dbo].[NewOrderDetail]
@OrderHeaderID int,
@ItemNumber varchar(50),
@ItemDescription varchar(50),
@QuantityOrdered int
AS
BEGIN
SET NOCOUNT ON;
INSERT INTO OrderDetails(OrderHeaderID, ItemNumber, ItemDescription, QuantityOrdered)
VALUES(@OrderHeaderID, @ItemNumber, @ItemDescription, @QuantityOrdered)
END
GO