5.
Improving your handler to check the last modified time
Because you can retrieve the
last modified time, you can modify your handler to check whether the
file has changed since you last downloaded it. If it has changed, you
can download another copy of the file. The following listing shows the
modified code for your handler.
Listing 2. Checking the last modified date
At
you get
the last modified time of the BLOB.
After you’ve gotten the
last modified time of the BLOB, then you check
whether you already have the file locally and whether the local file is
older than the server file. The final change that you need to make is
that you set the last write time of the local file to the last modified
time of the BLOB
.
So far, we’ve only looked at the
properties of a BLOB. Now let’s look at the other part of the BLOB data
that’s returned in a HEAD request: custom metadata.
6. Adding and
returning custom metadata
In listing 1,
we showed you how to view all the headers associated with a BLOB using a
console application. In that example, you returned all the standard
headers associated with a BLOB (last-modified, x-ms-request-id, and so on). If you want to associate some custom
metadata with a file, that metadata would also be displayed in the
response headers.
Displaying Custom
Metadata
Let’s modify the BLOB
podcast01.mp3 to include the author of the file (me) as custom metadata.
Figure 3
shows the HEAD response in
the same console application using the same code that we developed in listing 2.
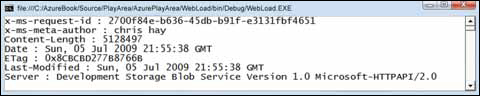
Note
that with the file podcast01.mp3, there’s a new header returned in the
response, called x-ms-meta-author, with the value chris hay. In this example, we returned the metadata from
the HEAD request, but it’s also available in
a GET request.
Setting Custom Metadata
If you need to set some custom
metadata, you can easily do this with the StorageClient library. The
following code sets the metadata for the file podcast01.mp3:
blob.Metadata.Add("Author", "Chris Hay");
blob.SetMetadata();
This code calls the UpdateBlobMetadata
method on the container, passing in a NameValueCollection of metadata that you want to store against the
BLOB.
The StorageClient
library automatically prefixes all metadata with the tag x-ms-meta-. We actually set the key as author in the metadata collection, but the response header
returned x-ms-meta-author. As a matter
of fact, the BLOB storage service will only allow you to set metadata
with the prefix x-ms-meta- and it
ignores any attempt to modify any other header associated with a BLOB.
Unfortunately, this means you can’t modify any standard HTTP header that
isn’t set by the BLOB storage service.
|
Scenarios for Using
Custom Metadata
The metadata support for
BLOBs allows you to have self-describing BLOBs. If you need to associate
extra information with a BLOB (for example, podcast author, recording
location, and so on), then this would be a suitable place to store that
data; you wouldn’t have to resort to an external data source. Any custom
attributes associated with a file (such as the author of a Word
document) could also be stored in the metadata.
Tip
If you need to be able to
search for metadata across multiple BLOBs, consider using an external
data source (for example, a database or the Table storage service),
rather than searching across the BLOBs.
Now that you know how the
upload and download operations operate under the covers, let’s return to
the final part of the uploading and downloading puzzle, namely, copying
BLOBs.