IYou used a file handler to
intercept MP3 requests from your website and to serve them from BLOB
storage rather than from your website. A more efficient approach would
be to check whether the file is already on your local filesystem. If the
file already exists, then you can just serve the file straight up. If
the file doesn’t exist, you can retrieve it from BLOB storage, store it
on the local filesystem, and then serve the file. All future requests
for the file won’t need to continually retrieve the file from BLOB
storage.
1. Using a local
cache
When you define Windows
Azure web roles, you can allocate a portion of the local filesystem for
use as a temporary cache. This local storage area allows you to store
semi-persistent data that you might use frequently, without having to
continually re-request or recalculate the data for every call.
You must be aware that this
local storage area isn’t shared across multiple instances and the
current instance of your web role is the only one that can access that
data. Figure 1 shows the distribution of BLOBs in local
storage across multiple instances.
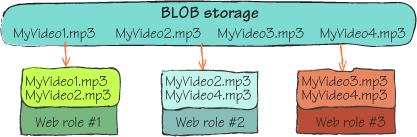
Because the load balancer evenly
distributes requests across instances, a user won’t always be served by
the same web role instance. Any data that you need persisted across
multiple requests (such as shopping cart or session data) shouldn’t be
stored in local storage and must be stored in a data store that all
instances can access.
2. Defining and
accessing local storage
You can define how much space you
require on your local filesystem in your service definition file. The FC
uses this information to assign your web role to a host with enough
disk space. The following code is how you would define that you need 100
MB of space to cache MP3 files:
<WebRole name="ServiceRuntimeWebsite">
<LocalResources>
<LocalStorage name="mp3Cache"
cleanOnRoleRecycle="true"
sizeInMB="100" />
</LocalResources>
</WebRole>
To access any of the files held in local storage, you
can use the following code to retrieve the location of the file:
LocalResource localCache =
RoleEnvironment.GetLocalResource("mp3Cache");
string localCacheRootDirectory = localCache.RootPath;
After you’ve retrieved the
root directory of local storage (RootPath),
you can use standard .NET filesystem calls to modify, create, read, or
delete files held in local storage.
Great! You’ve
configured your local storage area. Now you need to modify your HTTP
handler to use your local storage area, where possible.