In this section, we take a closer look at building applications with .NET Business Connector, including the following topics:
1. Data Types and Mappings
. NET Business Connector makes it easier to
develop managed applications that integrate with Dynamics AX by bridging
two programming environments: the managed .NET Framework environment
and the Dynamics AX X++ environment. Inevitably, some form of
translation is required when passing objects and data between these two
environments. Table 1 maps equivalent data types between the .NET Framework and Dynamics AX.
Table 1. Data Type Mappings
Dynamics AX Data Type | .NET Framework Data Type |
---|
String | System.String |
Int | System.Int32 |
Real | System.Double |
Enums | System.Enum
.NET Business Connector uses integers for enumerations. |
Time | System.Int
You must convert this value to Dynamics AX time format. The value is the number of seconds since midnight. |
Date | System.Date
You need to use only the date portion because time is stored separately in Dynamic AX. |
Container | AxaptaContainer |
Boolean (enumeration) | System.Boolean
Dynamics AX uses integers to represent Boolean values of true and false. |
GUID | System.GUID |
Int64 | System.Int64 |
The managed class methods in .NET Business
Connector explicitly support specific data types for parameters and
return values. Refer to the Microsoft Dynamics AX 2009 software
development kit (SDK) for more information.
2. Managed Classes
This
section provides an overview of the managed classes in .NET Business
Connector. You develop applications with .NET Business Connector by
instantiating and using the public managed classes described in Table 2.
Table 2. .NET Business Connector Managed Classes
Class Name | Description |
---|
Axapta | Provides
methods for connecting to a Dynamics AX system, creating Dynamics AX
objects (class objects, record objects, container objects, and buffer
objects), and executing transactions. |
AxaptaBuffer | Represents an array of bytes and provides methods for manipulating the buffer contents. AxaptaBuffer objects can be added to AxaptaContainer objects. |
AxaptaContainer | Provides methods for reading and modifying containers. |
AxaptaObject | The managed representation of an X++ class. Instance methods can be called through it. |
AxaptaRecord | Provides methods for reading and manipulating common objects (tables in the Dynamics AX database). |
Examples of how these classes are used in an application are provided in the following section.
3. Processing Requests and Responses
Much like any integration component, .NET
Business Connector processes requests and returns responses associated
with the use of the managed classes by applications across all the
established .NET Business Connector user sessions.
Request Processing
Figure 1 depicts the processing steps associated with a request made through the managed classes.
1. | A request is initiated by invoking a managed class.
|
2. | The request is received and marshaled across the transition layer (where .NET objects and data are converted from .NET to X++).
|
3. | The transition layer dispatches the request to the interpreter in .NET Business Connector.
|
4. | If
the request involves executing X++ code, this code is run either
locally or remotely on the AOS, depending on the directive associated
with the code.
|
5. | After the request is processed, a response is generated.
|
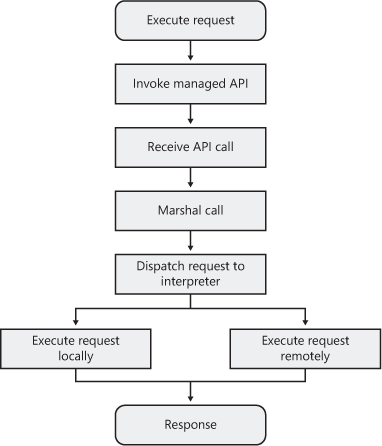
Response Processing
Figure 2 depicts the processing steps associated with generating a response.
1. | The active request processed by .NET Business Connector completes, successfully or unsuccessfully.
|
2. | The interpreter instantiates and dispatches the response to the transition layer.
|
3. | The transition layer marshals the response to the managed classes (converting objects and data from X++ to .NET).
|
4. | The response is returned to the caller, which is the application that initially invoked .NET Business Connector.
|
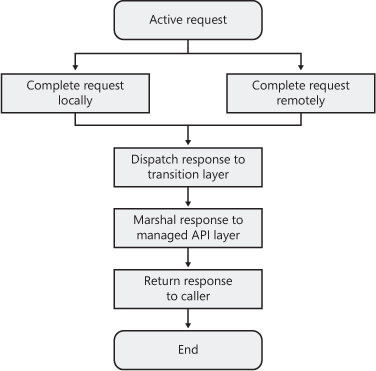
The main variation in the request and response
cycle is the location where the X++ code being invoked is executed. The
declaration associated with the X++ code controls this location. By
default, the X++ code runs where called—that is, from the interpreter
where it is invoked. If the client keyword is used, execution is forced on either .NET Business Connector or the Dynamics AX client. If the server keyword is used, the AOS executes the code.