You’ve
uploaded files and downloaded files in and out of your account. But you
don’t always want to transfer files outside your account; sometimes you
might want to take a copy of an existing file in the account.
Let’s return to the
podcasting example for a lesson on why you might want to do this. Let’s
say that during the conversion of a WMA file to MP3, you decide that you
don’t want to make the converted file immediately
available. In this case, your converted MP3 file would reside in a
private container that isn’t available to the general public. At a later
date, you decide to make the file available to the public by moving it
into your public downloads container. To do this, you copy your
converted file from one container to another. Figure 1 shows a file being copied from one container to another.
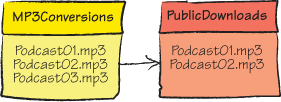
In figure 1, the BLOB isn’t being uploaded or downloaded; the BLOB
podcast01.mp3 is just being copied from the MP3Conversions container and
the copy is being placed in the Public-Downloads container.
Although you could do this
using a download followed by an upload, this would be much slower than
just doing an internal copy within the data center. Likewise, performing
an upload followed by a download would be incredibly slow and wasteful
of network resources if the calling client was based outside the Windows
Azure data center.
Listing 1
shows the code used to copy a very large filename podcast03.mp3 to the
PublicDownloads container from the MP3Conversions container via the REST
API.
Listing 1. Copying a BLOB between containers
As you can see in listing 1, the basics of making the HTTP request is pretty much the
same as any other REST request you’ve made. In this case, you’re setting
the destination container
as the
URI for the request, and you’re setting the request to be a PUT
(rather than a GET, HEAD, or POST).
At
you
set the location of the source BLOB to be copied. The header x-ms-copy-source is where you define the location of the file;
notice that we’re including the storage account name (silverlightukstorage),
the container (mp3conversions), and the BLOB name (podcast03.mp3)
in the header value.
1. Copying files
via the StorageClient library
Listing 1 uses the REST API directly to copy the BLOB, but you could make
this call by making the simpler StorageClient library call:
var sourceBlob = sourceContainer.GetBlobReference("podcast01.mp3");
var targetBlob = targeCcontainer.GetBlobReference("podcast01.mp3");
targetBlob.CopyFromBlob(sourceBlob);
This code makes the same call
as in listing 1, this time using the StorageClient
library instead of using the REST API directly.
Note
Although you can use the REST
API, using the StorageClient library is much easier. Save yourself a
lot of heartache and use the REST API only when necessary. Try to stick
to using the lovely StorageClient library.
The problem of copying
BLOBs is that you have to pay storage costs to the Microsoft bean
counters for keeping duplicate copies of the data. If you’re only
copying the BLOB to maintain some sort of version control, you should
consider snapshotting instead. A snapshot pins a version of your BLOB at
the date and time you created the snapshot. The snapshot is read only
(it can’t be modified) and can be used to revert to earlier versions of a
BLOB. Only changes made between versions of snapshots are chargeable.
To create a snapshot, you can
make the following call:
var snapshotBlob = blob.CreateSnapshot();
To retrieve a snapshot via the
REST API, you can use the following URI:
http://accountname.blob.core.windows.net/containername/blobname?snapshot=<DateTime>
You can also retrieve a
snapshot using the StorageClient library:
var snapshotBlob = container.GetBlobReference("blobname?snapshot=<DateTime>");
For more details
about snapshotting, go to http://msdn.microsoft.com/en-us/library/ee691971.aspx.
|