Each gesture will update a text label with information
about the gesture that has been detected. Pinch, rotate, and shake will
take things a step further by scaling, rotating, or resetting an image
view in response to the gestures.
Implementation Overview
This application, which we’ll name Gestures, will display a screen with four embedded views (UIView), each assigned a different gesture recognizer within the viewDidLoad
method. When you perform an action within one of the views, it will
call a corresponding method in our view controller to update a label
with feedback about the gesture, and depending on the gesture type,
update an onscreen image view (UIImageView), too.
The final application is shown in Figure 1.
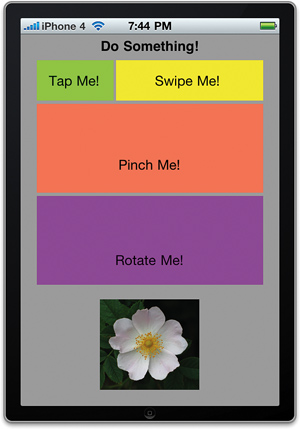
Setting Up the Project
Start Xcode and create a new View-Based iPhone application called Gestures. Next, open up the GesturesViewController.h interface file and add outlets and properties for four UIViews: tapView, swipeView, pinchView, and rotateView. These are the views to which we’ll attach gesture recognizers. Add two additional outlets and properties, outputLabel and imageView, of the classes UILabel and UIImageView, respectively. These will be used to provide feedback to the user.
Listing 1 shows the finished interface file.
Listing 1.
#import <UIKit/UIKit.h>
@interface GesturesViewController : UIViewController {
IBOutlet UIView *tapView;
IBOutlet UIView *swipeView;
IBOutlet UIView *pinchView;
IBOutlet UIView *rotateView;
IBOutlet UILabel *outputLabel;
IBOutlet UIImageView *imageView;
}
@property (nonatomic, retain) UIView *tapView;
@property (nonatomic, retain) UIView *swipeView;
@property (nonatomic, retain) UIView *pinchView;
@property (nonatomic, retain) UIView *rotateView;
@property (nonatomic, retain) UIView *outputLabel;
@property (nonatomic, retain) UIImageView *imageView;
@end
|
To keep things nice and neat, edit the implementation file (GesturesViewController.m) to include @synthesize directives after the @implementation line:
@synthesize tapView;
@synthesize swipeView;
@synthesize pinchView;
@synthesize rotateView;
@synthesize outputLabel;
@synthesize imageView;
Finish off these preliminary steps by releasing each of these objects in the dealloc method of the view controller:
- (void)dealloc {
[tapView release];
[swipeView release];
[pinchView release];
[rotateView release];
[outputLabel release];
[imageView release];
[super dealloc];
}
Adding the Image Resource
Before
you can create your application’s gesture-aware interface, you need to
add an image to the project. We will use this to provide visual feedback
to the user. Included in this hour’s project’s Images folder is
flower.png. Drag this file onto the Resources group for your project,
choosing to copy it to the project, if needed, as shown in Figure 2.