To keep things simple, we’ll return to the Hawaiian Shirt Shop website.
Over the next few sections, we’ll look at how we’d typically store
shirt data in a noncloud database. We’ll focus on the following:
How would we represent a shirt in C#?
How would we store shirt data in SQL Server?
How would we map and transfer data between the two platforms?
By understanding how
we’d represent our shirt data in typical solutions, we can then see how
this translates to the Table service.
1. How we’d normally represent an entity in C#
We defined a Hawaiian shirt using the following data transfer object (DTO) entity.
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public string Description { get; set; }
}
The Product entity class that we’re using to represent Hawaiian shirts contains three properties that we’re interested in (Id, Name, and Description).
We chose to keep the example simple by hardcoding the list of products
rather than retrieving it from a data store such as the Table service or
SQL Server. The following code is a hardcoded list of the three shirt
entities displayed in figure 1 (Red Shirt, Blue Shirt, and Blue Frilly Shirt).
var products =
new List<Product>
{
new Product
{
Id = 1,
Name = "Red Shirt",
Description = "Red"
},
new Product
{
Id = 2,
Name = "Blue Shirt",
Description = "A Blue Shirt"
},
new Product
{
Id = 3,
Name = "Blue Frilly Shirt",
Description = "A Frilly Blue Shirt"
},
};
In the preceding code, we
simply defined the list of products as a hardcoded list. Obviously this
isn’t a very scalable pattern—you don’t want to redeploy the application
every time your shop offers a new product—so let’s look at how you can
store that data using a non-Windows Azure environment, such as SQL
Server.
.2. How we’d normally store an entity in SQL Server
To store an entity in SQL Server, you first need to define a table where you can store the entity data. Figure 1 shows how the Products table could be structured in SQL Server.
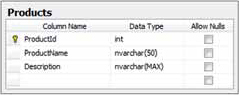
Figure 1
shows a table called Products with three columns (ProductId,
ProductName, and Description). In this table, ProductId would be the
primary key and would uniquely identify shirts in the table. Table 1 shows how the shirt data would be represented in SQL Server.
Table 1. Logical representation of the Products table in SQL Server
ProductId | ProductName | Description |
---|
1 | Red Shirt | Red |
2 | Blue Shirt | A Blue Shirt |
3 | Blue Frilly Shirt | A Frilly Blue Shirt |
In table 1
we’ve enforced a fixed schema in our SQL Server representation of the
Hawaiian shirts. If you wanted to store extra information about the
product (a thumbnail URI, for example) you’d need to add an extra column
to the Products table and a new property to the Product entity.
Now that we can represent
the Hawaiian shirt product as both an entity and as a table in SQL
Server, we’ll need to map the entity to the table.
3. Mapping an entity to a SQL Server database
Although you can manually
map entities to SQL Server data, you’d typically use a data-access layer
framework that provides mapping capabilities. Typical frameworks
include the following:
The following code maps the Products table returned from SQL Server as a dataset to the Product entity class using LINQ to DataSet.
var products = ds.Tables["Products"].AsEnumerable().Select
(
row => new Product
{
Id = row.Field<int>("ProductId"),
Name = row.Field<string>("ProductName"),
Description = row.Field<string>("Description")
}
);
In this example, we convert the dataset to an enumerable list of data rows and then reshape the data to return a list of Product entities. For each property in the Product entity (Id, Name, and Description) we map the corresponding columns (ProductId, ProductName, and Description) from the returned data row.
We’ve now seen how
we’d normally define entities in C#, how we’d represent entities in SQL
Server, and how we could map the entity layer to the database. Let’s
look at what the differences are when using the Table service.