We will now create an ASP.NET Web service (.asmx) to encapsulate our Web methods. The first Web method, GetPosting(), will return a serialized LightweightPosting
object and during serialization the properties and public fields will
be serialized and nested in the XML. The second Web method, SavePosting(), will accept a LightweightPosting and call the Save() method to update MCMS.
To create the ASP.NET Web service, perform the following steps:
1. | In Visual Studio .NET, right-click on the McmsAuthoringWebService project and select Add | Add Web Service.
|
2. | Name this Web service Authoring.asmx and click OK.
|
3. | Click on the Click Here link to switch to code view.
|
4. | Add the following namespaces to the file:
using System.Security.Principal;
using Microsoft.ContentManagement.Publishing;
|
5. | Delete the following code as we will not be needing it:
// WEB SERVICE EXAMPLE
// The HelloWorld() example service returns the string Hello World
// To build, uncomment the following lines then save and build the project
// To test this web service, press F5
// [WebMethod]
// public string HelloWorld()
// {
// return "Hello World";
// }
|
The GetPosting() Web Method
Now let’s define our GetPosting() Web method that will populate one of our custom LightweightPosting objects from MCMS. For GetPosting() to be accessible via a Web service it must have the [WebMethod] attribute. If the strPath parameter is an empty string we will initialize an empty LightweightPosting object so the complete object model is serialized.
/// <summary>
/// Gets a Lightweight posting and all the properties by path
/// </summary>
/// <param name="strPath">Path of the posting</param>
/// <returns>LightweightPosting</returns>
[WebMethod]
public LightweightPosting GetPosting(string strPath)
{
// Did we pass in a path?
if (strPath == "")
{
// Define a lightweight posting object
LightweightPosting _LightweightPosting = new LightweightPosting();
// Initialize the Custom Properties, Placeholders and Template
_LightweightPosting.CustomProperties =
new LightweightCustomPropertyCollection();
_LightweightPosting.Placeholders =
new LightweightPlaceholderCollection();
_LightweightPosting.Template = new LightweightTemplate();
// Create an empty placeholder
LightweightPlaceholder _placeHolder = new LightweightPlaceholder();
// Load an empty string into the Value property
_placeHolder.Value = _placeHolder.LoadXHTML("");
// Add the placeholder to the collection
_LightweightPosting.Placeholders.Add(_placeHolder);
// Return the posting object
return(_LightweightPosting);
}
else
{
// Declare a Posting object
Posting _Posting = null;
// Create the new application context
CmsApplicationContext cmsContext = new CmsApplicationContext();
// We need to get the identity of the current user
WindowsIdentity ident = System.Web.HttpContext.Current.User.Identity
as WindowsIdentity;
try
{
// Authenticate into update mode
cmsContext.AuthenticateUsingUserHandle(ident.Token,
PublishingMode.Update);
}
catch(Exception ex)
{
// Dispose of the CMS Context
cmsContext.Dispose();
throw new Exception("Failed to Authenticate user by handle. "
+ "The user may not have author permissions.", ex);
}
// Get the MCMS Posting object by path
_Posting = (Posting)cmsContext.Searches.GetByPath(strPath);
// Verify the posting was found
if (_Posting == null)
{
// Dispose the CMS Context
cmsContext.Dispose();
throw new Exception("The posting was not found at path '"
+ strPath + "'");
}
// Instantiate the lightweight posting passing
// in the MCMS posting to the constructor.
LightweightPosting _LightweightPosting = new LightweightPosting(_Posting);
// Dispose the CMS Context
cmsContext.Dispose();
// Return the lightweight posting
return(_LightweightPosting);
}
}
Configuring IIS Security
As we are using the current user’s Windows identity to authenticate the CmsApplicationContext, we should verify anonymous access to this Web service is disabled.
1. | In the web.config file, verify the authentication element is set to: <authentication mode=“Windows” />
|
2. | In the Internet Information Services (IIS) management console, right-click on the McmsAuthoringWebService virtual directory and select Properties.
|
3. | Select the Directory Security tab.
|
4. | Under Anonymous access and authentication control, select Edit.
|
5. | Uncheck Anonymous access and check Integrated Windows authentication.
|
6. | Click OK and close all opened dialogs.
|
7. | Close the IIS management console.
|
Testing the Web Service
To test it out, we can set the Web service as the start page for the project by right-clicking on authoring.asmx in Solution Explorer, and selecting Set As Start Page. Then start without debugging by pressing Control-F5 or selecting Start Without Debugging from the Debug menu.
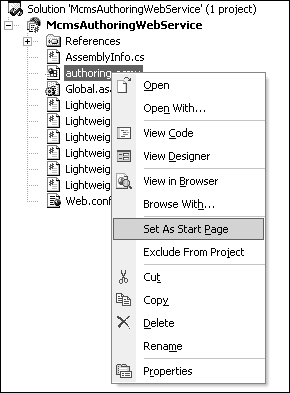
We should now see the ASP.NET Web service test page, listing GetPosting as the single available Web method:
If you now click on GetPosting, you will see a textbox for the parameter strPath. Enter /channels/www.tropicalgreen.net/PlantCatalog/AloeVera into the box and click Invoke.
You will now see the serialized view of the LightweightPosting object and all its serializable properties:
The SavePosting() Web Method
Let’s create another Web method, SavePosting(), which will accept a LightweightPosting object as a parameter and save its contents to MCMS. Add the following code to the authoring.asmx.cs file and rebuild the solution:
/// <summary>
/// Save a lightweight posting object to MCMS
/// </summary>
/// <param name="_Posting">LightweightPosting object to save</param>
[WebMethod]
public void SavePosting(LightweightPosting _Posting)
{
// Save the posting
_Posting.Save();
}
The SavePosting() Web method cannot be tested from the browser as we did for GetPosting() as it requires a complex datatype (LightweightPosting) as a parameter. We will test SavePosting() via the InfoPath form we will create shortly.