Maintaining Placeholder Collections
Let’s now create a collection class to hold LightweightPlaceholders that we’ll call LightweightPlaceholderCollection. The LightweightPlaceholderCollection will be used as postings may have more than one placeholder defined.
1. | In Visual Studio .NET, create a new class, LightweightPlaceholderCollection, and add the MCMS Publishing and Placeholders namespaces to it. Also change the class to extend System.Collections.CollectionBase:
using System;
using Microsoft.ContentManagement.Publishing;
using Microsoft.ContentManagement.Publishing.Extensions.Placeholders;
namespace McmsAuthoringWebService
{
/// <summary>
/// Summary description for LightweightPlaceholderCollection.
/// </summary>
public class LightweightPlaceholderCollection
: System.Collections.CollectionBase
{
. . . code continues . . .
}
}
|
2. | As LightweightPlaceholderCollection is a strongly typed collection, the default property will be a LightweightPlaceholder:
/// <summary>
/// The indexed LightweightPlaceholder
/// </summary>
public LightweightPlaceholder this[int index]
{
get
{
// Cast the list item to a LightweightPlaceholder
return(this.InnerList[index] as LightweightPlaceholder);
}
set
{
this.InnerList[index] = value;
}
}
|
3. | We now define the Add() method that will add a LightweightPlaceholder to the collection:
/// <summary>
/// Add an item to the collection
/// </summary>
/// <param name="item">The LightweightPlaceholder to be added</param>
public void Add(LightweightPlaceholder item)
{
this.InnerList.Add(item);
}
|
4. | The LightweightPlaceholderCollection will be populated by loading a Microsoft.ContentManagement.Publishing.Posting object, so let’s create the Load() method.
For the purposes of this example we are only maintaining placeholders of type HtmlPlaceholder. The code could be easily extended to maintain different types of placeholders.
/// <summary>
/// Load the lightweight Placeholder collection from a posting.
/// </summary>
/// <param name="_Posting">Posting to be loaded from</param>
public void Load(Posting _Posting)
{
if (_Posting != null)
{
// Loop through the Placeholders
foreach (Placeholder _Placeholder in _Posting.Placeholders)
{
// Check if the custom Placeholder is an HtmlPlaceholder
if (_Placeholder is HtmlPlaceholder)
{
// Instantiate a lightweight Placeholder and
// add it to the collection
this.Add(new LightweightPlaceholder(_Placeholder));
}
}
}
}
|
5. | To save the content of all the placeholders in the collection, we will create a Save() method that iterates through all the placeholders and calls Save() for each:
/// <summary>
/// Save the collection of placeholders
/// </summary>
/// <param name="_Posting">Posting to save the placeholder values to</param>
public void Save(Posting _Posting)
{
// Loop the Placeholders in the collection
foreach (LightweightPlaceholder _LightweightPlaceholder in this)
{
// Save the Placeholder against the posting
_LightweightPlaceholder.Save(_Posting);
}
}
|
Now we have defined our
classes for maintaining custom properties and placeholders, and for
storing template definition data, the McmsAuthoringWebService project should look as in the figure overleaf:
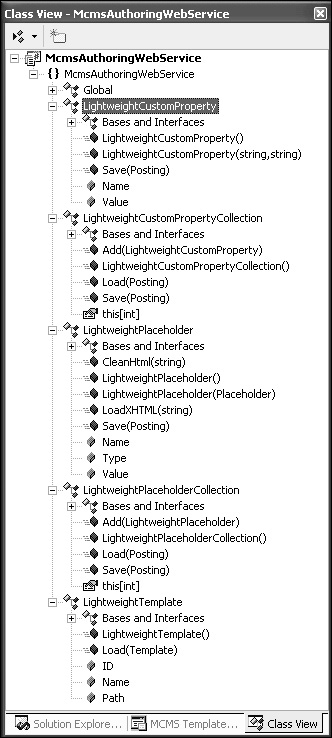