Microsoft has provided two
very extendable frameworks: the Microsoft SharePoint and the Microsoft
CRM APIs. The two systems have a lot of their APIs exposed (the details
can be found on the MSDN site), which can allow developers to automate
business processes for nearly every business permutation. (See, for
example, Figure 1.)
The APIs for both systems enable developers to accomplish numerous
things. For example, an application can be created to trigger a
forms-based workflow inside SharePoint when an attribute changes in
Microsoft Dynamics CRM. The possibilities are endless via custom
development.
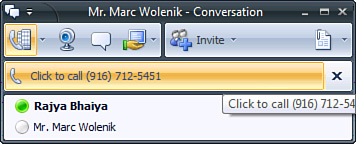
The Microsoft Dynamics CRM (http://msdn.microsoft.com/en-us/library/bb928212.aspx)
SDK contains architectural information about the entity and security
model. The SDK also contains sample code for the following features:
The Microsoft SharePoint APIs (http://msdn.microsoft.com/en-us/library/ms441339.aspx) enable developers to create modules for the following components:
The server-side object model allows
developers to interact with the MOSS and WSS components directly (for
example, populating data in Microsoft Dynamics CRM after a series of
approvals on the data stored in a SharePoint list).
The web services definition is the recommended mode of communication with Dynamics CRM and SharePoint.
The Collaborative Application Markup Language (CAML) is an optimized way of retrieving data from SharePoint.
You can use workflows to encapsulate business processes that can be attached to SharePoint lists in Windows SharePoint services.
The
Create Custom Field Types option is a way to store custom data types
inside SharePoint for further integration (for example, creating a data
type for a geographic information system (GIS), also known as a maps
integration).
Search APIs provide direct access to the query objects and Query web service to retrieve search results.
To expedite web part development, Microsoft has provided a starter kit for the following development environments:
Open Visual Studio and create a new project using
the WebPart template. This will create a basic web part shell, with a
deployment package. The basic WebPart template has the following code
inside it:
using System;
using System.Runtime.InteropServices;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Serialization;
using Microsoft.SharePoint;
using Microsoft.SharePoint.WebControls;
using Microsoft.SharePoint.WebPartPages;
namespace WebPart1
{
[Guid("e499bfed-b3d8-4bcf-9d03-642337145800")]
public class WebPart1 : System.Web.UI.WebControls.WebParts.WebPart
{
public WebPart1()
{
}
protected override void CreateChildControls()
{
base.CreateChildControls();
// TODO: add custom rendering code here.
// Label label = new Label();
// label.Text = "Hello World";
// this.Controls.Add(label);
}
}
}
Using the basic template, you can just enter the
following lines of code inside the Render function. The following lines
of code will show a list of accounts, where the account name starts with
ExtraTeam (as shown in Figure 2):
CrmSdk.CrmAuthenticationToken authToken = new CrmAuthenticationToken();
authToken.OrganizationName = "MicrosoftCRM";
CrmSdk.CrmService crmService = new CrmSdk.CrmService();
crmService.Credentials = System.Net.CredentialCache.DefaultCredentials;
crmService.CrmAuthenticationTokenValue = authToken;
QueryExpressionHelper qeHelper = new
QueryExpressionHelper(EntityName.account.ToString());
qeHelper.Columns.AddColumn("name");
qeHelper.Criteria.Conditions.AddCondition("name", ConditionOperator.Like, "ExtraTeam%");
BusinessEntityCollection accountsRetrieved =
service.RetrieveMultiple(qeHelper.Query);
foreach (account accountItem in accountsRetrieved.BusinessEntities)
writer.Write(accountItem.name + "<BR>");
Note
It is recommended to separate the application into separate functions and classes based on the requirements.